Python多进程1、在主进程中创建子进程python为我们提供了Process类,用于创建进程。使用Process类前,需要从multiprocessing模块引入。与Thread类的实例化方法类似,Process类实例化需要传递几个参数:target表示目...

Python多进程
1、在主进程中创建子进程
python为我们提供了Process类,用于创建进程。使用Process类前,需要从multiprocessing模块引入。与Thread类的实例化方法类似,Process类实例化需要传递几个参数:target表示目标函数、args表示目标函数的参数。
from multiprocessing import Process
import os
def coding(language):
print('I\'m coding %s ...' % language)
def music():
print('I\'m listening music ...')
if __name__ == '__main__':
print('parent process %s is running...' % os.getpid())
p1 = Process(target=coding, args=('Python',))
p2 = Process(target=music)
p1.start()
p2.start()
p1.join() #等待子进程结束
p2.join()
print('child process stop')
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
程序的运行结果如下:
2、进程池
如果要大量地创建进程,就要用到进程池Pool
from multiprocessing import Pool
from time import ctime
import os, time
def child_process(id):
time.sleep(1)
print('child process %s is running at ' % id, ctime())
if __name__ == '__main__':
print('parent process %s is running...' % os.getpid())
p = Pool() # 注意这里没有传进去参数
for i in range(11):
p.apply_async(child_process, args=(i,))
p.close()
p.join()
print('child process stop')
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
当Pool()构造函数没有传进去参数时,默认进程池的大小是CPU的核数,这里我的CPU是4核的。所以进程是以4个为1组运行的
当然,Pool也可以指定进程池的大小,把Pool()改为Pool(2),则进程以2个为1组运行
3、进程间通信
multiprocessing提供了Queue和Pipe实现进程间的通信
from multiprocessing import Process, Queue
import os
# 一个进程写数据
def write(q):
for i in range(100):
print('写入', i)
q.put(i)
# 另一个进程读数据:
def read(q):
while True:
value = q.get(True)
print('读出', value)
if __name__ == '__main__':
print('parent process %s is running...' % os.getpid())
# 在父进程中创建Queue,并传给各个子进程:
q = Queue()
pw = Process(target=write, args=(q,))
pr = Process(target=read, args=(q,))
pw.start()
pr.start()
pw.join()
pr.terminate() # 强行终止pr进程
print('child process stop')
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
程序运行的结果如下(只截了部分图):
我把Pipe进程间通信的代码,也粘在下面:
from multiprocessing import Process, Queue, Pipe
import os
# 一个进程写数据
def write(p):
for i in range(100):
print('写入', i)
p.send(i) # send 方法
p.close()
# 另一个进程读数据:
def read(p):
while True:
value = p.recv() # recv方法
print('读出', value)
if __name__ == '__main__':
print('parent process %s is running...' % os.getpid())
# 父进程创建Pipe,并传给各个子进程:
pipe = Pipe()
pw = Process(target=write, args=(pipe[0],))
pr = Process(target=read, args=(pipe[1],))
pw.start()
pr.start()
pw.join()
pr.terminate() # 强行终止
print('child process stop')
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
Python除了多进程外,多线程也经常被用到,有关多线程,参见:
http://blog.csdn.net/u010429424/article/details/76100149
多进程与多线程的对比
多进程的优点在于稳定性高,一个子进程的崩溃不影响主进程和其他子进程。但是创建进程的代价较大,os能够运行的进程数量也是有限的,这一点上看,多线程有一定的优势。
多线程速度比多进程快些,但是由于线程之间共享进程内存,一个线程崩溃后,会使整个进程崩溃,因此多线程的稳定性较差。
要实现多任务,我们往往会创建一个主进程和子进程,主进程分配任务给子进程,由子进程完成任务。 当然,创建主线程和子线程也可以。
加油哦~~
本文标题为:Python多进程
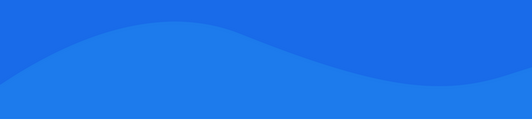
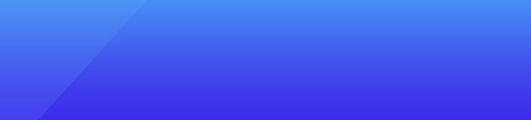
基础教程推荐
- Python爬取当网书籍数据并数据可视化展示 2023-08-11
- Python基础学习之函数和代码复用详解 2022-09-02
- centos系统 anaconda3(python3)安装pygrib 2023-09-04
- 基于Python实现股票数据分析的可视化 2023-08-04
- ubuntu 18 python3.6 的安装与 python2的版本切换 2023-09-03
- Centos7下安装python环境 2023-09-04
- CentOS 7.5 安装 Python3.7 2023-09-03
- 四步教你学会打包一个新的Python模块 2022-10-20
- python的环境conda简介 2022-10-20
- Python 中 Elias Delta 编码详情 2023-08-08