我正在尝试使用Tkinter GUI来启动子进程并将其stdout / stderr输出显示到Text小部件.最初,我认为通过设置“sys.stdout = text_widget”可以很容易地将sys.stdout重定向到Text小部件,但似乎没有.它出现错误:“文本实...

我正在尝试使用Tkinter GUI来启动子进程并将其stdout / stderr输出显示到Text小部件.最初,我认为通过设置“sys.stdout = text_widget”可以很容易地将sys.stdout重定向到Text小部件,但似乎没有.它出现错误:“文本实例没有属性’flush’”.
我在网上查了一下,得到了一些解决方案,比如使用Queue与子进程进行通信.但是,由于我的特殊要求,它们都不适合我的情况:
>最好通过“multiprocessing.Process”启动子进程,因为使用共享变量会更容易,这使得子进程解决方案可用.
>子进程的代码已经存在,里面有很多“打印”,所以我不想将它们修改为“Queue.put()”之类的东西.
在这种情况下,任何人都可以找到一个获得“multiprocessing.Process”的“打印”输出并显示到Tkinter文本的解决方案吗?非常感谢!
我的案例的示例代码如下:
import sys
import time
from multiprocessing import Process
from Tkinter import *
def test_child():
print 'child running'
def test_parent():
print 'parent running'
time.sleep(0.5)
Process(target=test_child).start()
def set_txt(msg):
gui_txt.insert(END, str(msg))
gui_txt.see(END)
if __name__ == '__main__':
gui_root = Tk()
gui_txt = Text(gui_root)
gui_txt.pack()
gui_btn = Button(gui_root, text='Test', command=test_parent)
gui_btn.pack()
gui_txt.write = set_txt
sys.stdout = gui_txt
gui_root.mainloop()
解决方法:
仍然可以使用队列而不必删除所有打印语句.您可以使用依赖于进程的stdout重定向来执行此操作.下面的解决方案使用Queue子类来模仿stdout.然后,该队列由一个线程监视,该线程查找被泵入文本小部件的新文本.
import sys
import time
from multiprocessing import Process
from multiprocessing.queues import Queue
from threading import Thread
from Tkinter import *
# This function takes the text widget and a queue as inputs.
# It functions by waiting on new data entering the queue, when it
# finds new data it will insert it into the text widget
def text_catcher(text_widget,queue):
while True:
text_widget.insert(END, queue.get())
# This is a Queue that behaves like stdout
class StdoutQueue(Queue):
def __init__(self,*args,**kwargs):
Queue.__init__(self,*args,**kwargs)
def write(self,msg):
self.put(msg)
def flush(self):
sys.__stdout__.flush()
def test_child(q):
# This line only redirects stdout inside the current process
sys.stdout = q
# or sys.stdout = sys.__stdout__ if you want to print the child to the terminal
print 'child running'
def test_parent(q):
# Again this only redirects inside the current (main) process
# commenting this like out will cause only the child to write to the widget
sys.stdout = q
print 'parent running'
time.sleep(0.5)
Process(target=test_child,args=(q,)).start()
if __name__ == '__main__':
gui_root = Tk()
gui_txt = Text(gui_root)
gui_txt.pack()
q = StdoutQueue()
gui_btn = Button(gui_root, text='Test', command=lambda:test_parent(q),)
gui_btn.pack()
# Instantiate and start the text monitor
monitor = Thread(target=text_catcher,args=(gui_txt,q))
monitor.daemon = True
monitor.start()
gui_root.mainloop()
本文标题为:Python多处理将子进程的stdout重定向到Tkinter Text
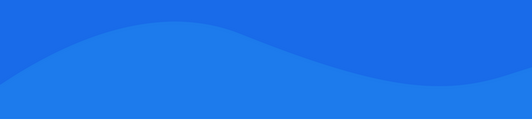
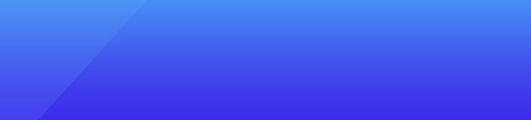
基础教程推荐
- Python-如何读取Windows“媒体创建”日期(而非文件创建日期) 2023-11-13
- Pygame库200行代码实现简易飞机大战 2023-08-04
- python – ‘使用postgres和Gunicorn Nginx作为反向代理的Django app中的类型inet’db错误的输入语法无效 2023-11-15
- Python yield 关键词, 2023-08-04
- Python+OpenCV实战之实现文档扫描 2022-10-20
- API例子:用Python驱动Firefox采集网页数据 | 一起学习Python网络爬虫 2023-09-04
- 在windows 怎么安装Python2.7 的 PIL包 2023-09-03
- Python函数进阶与文件操作详情 2022-09-02
- python FastApi实现数据表迁移流程详解 2022-08-30
- Python--day39--进程池原理及效率测试 2023-09-04